In this article, we will help you understand substrings in Java. We will not only give you a theoretical explanation but also give you real code examples to help you visualize. We will teach you how to create substrings and help you find substrings inside a string.
But before learning them, we have to have the basics of substrings.
What are strings and substrings?
In the context of Java, a string represents a sequence of characters. Every string in Java is an object. A string in Java can contain characters, symbols, and even whitespace. On the other hand, a substring in Java is a portion or a subset of a Java string.
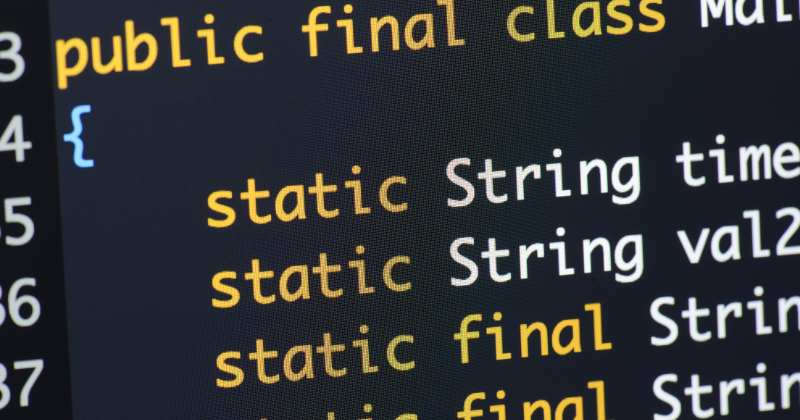
For example, “Geek” is a substring of “GeekFlare”. Substrings help you get a specific part of a string.
If you have the name “John Doe” and you want only the first name “John”, you can easily get it with substrings. Moreover, considering you have a list of names “John, Jack, Jolly” and you want to find out if “John” is in it, you can also do it with substrings. These are only mere examples. We can use substrings in various operations once we understand them.
As we are now aware of the concept of substrings in Java, now, let’s get to know how to create and work with substrings in Java.
Extraction of a Substring
#1. Using the ‘substring()’ method
The ‘substring()’ method lets us create substrings very easily. It takes up to two parameters as input – startIndex or both startIndex and endIndex and returns us the substring we want.
Depending on the number of parameters, we can use it in two ways. Now, let’s get to learn about them in detail.
substring(int startIndex)
To start, we can use the method in ‘substring(startIndex)’ form. Here, this method takes an integer value as input, where the input is the starting position of the substring. It returns a string starting from the provided start index till the end of the original string.
As an example, let’s look at the following code:
public class Substrings{
public static void main(String args[]){
String str="GeekFlare";
System.out.println("Given String: " + str);
System.out.println("Substring: " +str.substring(4)); //index of strings start from 0
}
}
OUTPUT:
Given String: GeekFlare
Substring: Flare
From the output, we see for the input string is “GeekFlare”, and the return value is the substring “Flare”. It makes a substring from the given index (4), that is, from position 5 to the end of the string.
substring(int startIndex, int endIndex)
This is another way to use the substring method of the String class. We can pass two integers to the substring method. A start index and an end index. To use this, we have to use the method in the ‘substring(startIndex,endIndex)’ format.
To understand it further, let’s look at some example code:
public class Substrings{
public static void main(String args[]){
String str="GeekFlareFans";
System.out.println("Given String: " + str);
System.out.println("Substring: " +str.substring(4,9)); //You get a substring starting from index no. 4 to index 8.
}
}
OUTPUT:
Given String: GeekFlareFans
Substring: Flare
As we can see, given the string “GeekFlareFans”, it outputs the substring “Flare”. We gave the start index of 4 and the end index of 9. It starts from the element with an index of 4 and ends before 9. We have to note that it does not print the element with the end index. It gives us a substring that includes all elements till the end index but excludes the element with the end index.
#2. Using the ‘split()’ method
The ‘split()’ is another method of the String class in Java that helps us create a substring. It is useful when multiple pieces of information are stored inside one string with a common separator character.
The syntax mentions the term “regex” which may seem a little intimidating to you, so let’s understand what regex is before proceeding. Regex is the short term for “Regular Expressions”. A regex is a sequence of characters that describes a pattern inside a string or text. In the context of the split method, the regex is our separator.
The ‘split()’ method can take up to two variables as input, they are a regex string and a limit integer. The regex is the separator, which, when found, results in splitting the original string into 2 parts – the part before the regex and the part after the regex.
For example, assume you are trying to split the string “abcdef” with “bcd” as regex. We would get the substrings “a” and “ef” as the result.
The method returns an array with the separated strings. We can either specify only the regex or both the regex and the limit. Let’s get to know about the multiple ways to call this method one by one.
split(String regex)
The first method receives only the regex string in the ‘split(regex)’ format. It doesn’t have a limit variable; therefore returns all the separated substrings in an array.
Let’s get a clear understanding with some code:
public class Substrings{
public static void main(String args[]){
String str="Geek%Flare";
String[] substrings=str.split("%");
System.out.println("Given String: " + str);
System.out.println("First Substring: " + substrings[0]);
System.out.println("Second Substring: " + substrings[1]);
}
}
OUTPUT:
Given String: Geek%Flare
First Substring: Geek
Second Substring: Flare
As we observe from the code, the given string has a separator regex “%”. It does not have to be a single character, it can be any string with any number of characters. The ‘split()’ method ignores this regex and gives us all the strings that were separated by this regex. The substrings are stored inside an array.
In the code, the given string is “Geek%Flare”. So, we get an array with two elements in it, which are “Geek” and “Flare”. We later accessed them with their respective indices, which are 0,1 respectively, and we printed “Geek” and “Flare” to the console.
Here we should also note that if no parameters are passed to the method, it will simply throw an error. But if we give an empty string (“”) as a regex, we will get every individual character as a substring. Let’s look at the example to visualize.
import java.util.Arrays;
public class Substrings{
public static void main(String args[]){
String str="Geek%Flare";
String[] substrings=str.split("");
System.out.println(Arrays.toString(substrings));
}
}
OUTPUT:
[G, e, e, k, %, F, l, a, r, e]
From the example, it is apparent as the regex parameter is an empty string, it returns all the characters as separate substrings, and we can clearly see this by printing the output array of the ‘split()’ method.
split(String regex,int limit)
With the second variant of this method, we get more control over the output, and we can further fine-tune the output of the ‘split()’ method. Here, the ‘split()’ method takes two variables as input. In this case, along with the regex, we also give a limit parameter in the specified format of ‘split(regex, limit)’.
The ‘limit’ is the number of resulting strings that are output. Depending on the value of the limit, there can be three possibilities:
Case 1: If limit>0, the resulting array would contain the output, but it would apply the split (limit-1) times at most. Here, the resulting array would not contain more elements than the specified limit, and all the leftover string that isn’t split would be stored as it was. Let’s make it easier to understand with some code.
import java.util.Arrays;
public class Substrings{
public static void main(String args[]){
String str="Geek%Flare%is%the%best";
String[] substrings=str.split("%",2);
System.out.println(Arrays.toString(substrings));
}
}
OUTPUT:
[Geek, Flare%is%the%best]
See in the output how there are only two elements in the result array which is the number given in the limit parameter. Also, notice that the split is applied only one time, so (limit-1) times.
However, if the regex is there two times in a row (“%%”), it would have empty substrings. Look at this bit of code to understand it better.
import java.util.Arrays;
public class Substrings{
public static void main(String args[]){
String str="Geek%Flare%is%%the%best%%%";
String[] substrings=str.split("%",5);
System.out.println(Arrays.toString(substrings));
}
}
OUTPUT:
[Geek, Flare, is, , the%best%%%]
Basically, if “%” is followed by another “%” or the end of the string, it transforms into an empty substring.
Case 2: If limit<0, the split action would be applied as many times it is possible with no limit to the array size, but the array would contain empty substrings if the regex is there two times in a row (“%%”).
import java.util.Arrays;
public class Substrings{
public static void main(String args[]){
String str="Geek%Flare%is%%the%best%%%";
String[] substrings=str.split("%",-1);
System.out.println(Arrays.toString(substrings));
}
}
OUTPUT:
[Geek, Flare, is, , the, best, , , ]
From the output, it is apparent that the split is applied as many times as possible, and also there are empty substrings present.
Case 3: If limit=0, the split action would also be applied as many times as possible, but here all the empty substrings at the end of the string would be discarded from the array.
import java.util.Arrays;
public class Substrings{
public static void main(String args[]){
String str="Geek%Flare%is%%the%best%%%";
String[] substrings=str.split("%",0);
System.out.println(Arrays.toString(substrings));
}
}
OUTPUT:
[Geek, Flare, is, , the, best]
We can see that the output is quite similar between when limit=-1 and when limit=0, but there are no trailing empty substrings. In other words, the empty substrings at the end of the substring array are ignored.
Also note that if the regex is not present in the string, it returns the entire original string as the result.
Find if a String Contains a Substring
Besides creating substrings from existing strings, we can also specify a substring and find out if that substring is existent inside a string. This is a quick and easy way to query a substring, and this is useful in many use cases. But how do we do it? Various methods can help us achieve this. Let’s go through them one by one.
Using ‘contains()’ method:
We can very easily find the existence of a substring with the ‘contains()’ method. This method of the String Class takes a string as input, which is our substring, and returns a boolean value checking if the substring is inside the string or not. This method can be used inside if-else blocks, unary operators, and various other places to implement complex logic.
Let’s get to know about this method a little more.
public class Substrings{
public static void main(String args[]){
String str="GeekFlare";
System.out.println("Does it contain Flare? \n"+ str.contains("Flare"));
}
}
OUTPUT:
Does it contain Flare?
true
The code checks the string “GeekFlare” for the word “Flare”, and on finding it successfully, it returns a boolean “true”, therefore confirming the existence of the substring.
public class Substrings{
public static void main(String args[]){
String str="GeekFlare";
System.out.println("Does it contain Flare? \n"+ str.contains("Flare1"));
}
}
OUTPUT:
Does it contain Flare?
false
From the example, we understand if the substring is not inside the string, the method will return false to signify its non-existence. So we can be easily sure if the substring exists.
Finding the Position of a Substring
#1. Using ‘indexOf()’:
The ‘indexOf()’ method can be used to find the existence of a substring and as well as find its index. The method takes as input a string or a character and gives us the position of its first occurrence. But it is only able to give us the index of the first occurrence and can’t confirm if other occurrences of this exist. Another thing to note here, if the substring doesn’t exist, the method returns -1.
So let’s explore this method a little more.
public class Substrings{
public static void main(String args[]){
String str="GeekFlareGeekFlare";
System.out.println("Index of Flare: "+ str.indexOf("Flare"));
}
}
OUTPUT:
Index of Flare: 4
Here in the example, the first occurrence of the substring “Flare” starts from index 4 in the string “GeekFlareGeekFlare”. So, as expected, the function returned the index.
#2. Using ‘lastIndexOf()’:
‘lastIndexOf()’ is very similar to ‘indexOf()’. Both of these methods take as input the substring and return the index of its position. It even has the same return value when it can’t find the substring in the specified string. Both of these methods return -1 for an unsuccessful find.
But while ‘indexOf()’ returns the index of the first occurrence of the substring, ‘lastIndexOf()’ returns the last occurrence.
Let’s see it in action through code:
public class Substrings{
public static void main(String args[]){
String str="GeekFlareGeekFlare";
System.out.println("Last index of Flare: "+ str.lastIndexOf("Flare"));
}
}
OUTPUT:
Last index of Flare:13
Observing this output, we understand that the method ‘lastIndexOf()’ behaves as expected and we get the index of the last occurrence of the substring “Flare” in the string “GeekFlareGeekFlare”.
FAQs
If there are multiple regex instances of the regex string in the main string one after another(“Hello%%Hi”, where regex is “%”), the ‘split()’ method considers the first instance as a break character, and the rest gives the output of an empty string. To mitigate this, we can specify the limit parameter as 0. Hence it will give only non-empty strings as output.
No, ‘indexOf()’ doesn’t return indexes of all the instances of a substring. With ‘indexOf()’, we get an integer return value containing the index of the first occurrence of the substring. But if it can’t find the substring, the method will return -1.
If the given start index and the end index don’t exist in the string, the compiler will throw an error. The error should have “java.lang.StringIndexOutOfBoundsException: ” and it will simply not execute.
Conclusion
In this article, we discussed various methods and essentials to get started with substrings. We discussed creating a substring and checking the existence of a substring within a string. This will give you a better understanding of how to work with substrings. Follow the examples and practice more to get a thorough understanding of substrings.
Next, you may check our list of Java interview questions.