When writing a program, you rarely write all the code in one go and only run your code at the end. Instead, you are most likely to write some code, run the program, write some more, and repeat until you are done.
Effectively you will divide and conquer your program by focusing on some essential parts and omitting others, then returning to fill in the blanks. To do this effectively in Python, you must use the pass statement, this article’s subject.
What Is the ‘Pass’ Statement?
Unlike most keywords that tell the interpreter to do something, the pass statement is unique because it tells the interpreter to do nothing. It is used inside blocks.
Blocks in Python are lines of code indented under functions, classes, or control flow statements. Control flow statements are statements that change the normal top-to-bottom execution of a program by either skipping over code using conditionals or repeating the execution of code using loops. The common control flow keywords in Python are if, for, and while.
Need of ‘Pass’ Statement
I explained earlier that the pass statement is a statement that does nothing. But why would we need a statement that does nothing when building a program that does something? In this section, I will explain the use cases for the pass statement.
#1. Placeholder for Future Implementation
As mentioned in the introduction, when coding, it is helpful to divide and conquer when writing your program. For example, when writing a function, you might want to create a function header and come back to write the function body later.
But the Python interpreter will yell at you if you try to run the code. This is because Python expects the body of a function, or any code block, to contain at least one statement. Here’s an example function I have in a Python file:
def my_function():
# Will write the code later
# For now there are no statements
Now if I try to execute the code, I will get a syntax error that says something like this:
File "<ipython-input-1-58189b01193e>", line 3
# For now there are no statements
^
SyntaxError: incomplete input
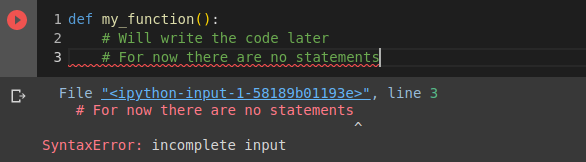
Our divide-and-conquer strategy won’t work if we write everything beforehand, but Python needs us to write at least something. The pass statement helps in that regard. It is technically a statement, so if you included it in a block, Python would not yell at you. And yet it does nothing, so you do not have to worry about the function logic. Here’s the previous function with the pass statement.
def my_function():
# Will write the code later
# For now we only have the pass statement
pass
Now if we run the code, we do not get errors.

You can also call the function to confirm it does nothing.
Essentially this is the main use case of the pass statement in Python – as a placeholder for empty code blocks. It does not have to be just functions but works with conditionals, loops, and classes.
#2. Implementing Abstract Classes and Interfaces
The pass statement can also be used to implement an abstract class. An abstract class is a class that implements abstract methods, and an abstract method is a method that is defined but not implemented. This is similar to the use case defined above, where we used the pass statement as a placeholder. However, unlike the previous case where the pass statement was only temporary, in this case, it is permanent.
Abstract classes are base classes that define the methods the superclasses inheriting from them should implement. Abstract classes do not implement any of the logic; they define the methods that make up the classes inherited from them. As a result, you do not instantiate a base class directly.
You use the @abstractmethod decorator and ABC class defined in the abc package to create abstract classes in Python. If you are unfamiliar with or forgot about decorators, here’s an article on decorators in Python.
As I was saying, the abc package defines the @abstractmethod decorator and ABC class, which we will use as follows:
from abc import (abstractmethod, ABC)
class BasicClass(ABC):
@abstractmethod
def basic_method(self):
# Basic method is a method that any superclass of BasicClass should implement
# So we simply define it here and not implement it
# So in the body we use the pass statement so its valid code
pass
BasicClass inherits from the ABC class. In addition, we have the @abstracmethod decorator defined around basic_method. Now, you can inherit from this class and implement basic_method.
class DerivedClass(BasicClass):
def basic_method(self):
print("Hello from the defined basic method")
You can then instantiate this class and test run the basic_method.
my_object = DerivedClass()
my_object.basic_method()
When you run this, you should be able to get the message written on the screen.
Hello from the defined basic method
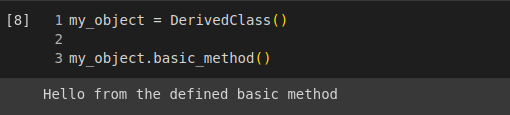
#3. To Do Nothing with Caught Exceptions
When the program encounters an error in Python, it raises an exception. Exceptions are disruptive as they cause the program to crash and stop execution. However, you can catch and handle exceptions to prevent the program from crashing. You can use the pass statement if you do not want to do anything in particular to handle the error. Here’s an example:
try:
# code that will definitely raise an exception
raise Exception('Some exception')
except:
pass
If you run the above code, nothing happens. This is because the exception is raised and handled by the code in the except block. But this code does nothing, so nothing happens. Normally, with exceptions, you would want to log them or handle them gracefully. But if you want to do nothing, that is how you would do it.
How Does the Pass Statement Differ from Break and Continue?
Other keywords you might encounter are break and continue. In short, I will explain what they do so you may see how they differ from the pass statement.
Break Statement
The break statement is used to break out of a loop. Every other subsequent iteration of the loop is then canceled. For example, suppose you were writing a linear search function. When the element is found, there is no reason to continue iterating to the end of the list. It would make sense to break out of the loop. So you would use the break statement. Here is an example linear search function:
def linear_search(values, search_value):
for i in range(len(values)):
if values[i] == search_value
print("Value found at index", i)
break
Continue Statement
The continue statement is used to skip the current iteration in the loop. If you are iterating over a list of numbers, when your program encounters a continue statement, it will halt the current iteration and skip over to the next iteration. The loop continues running. The following is an example function that uses the continue statement. The program doubles all odd numbers, so a list only contains even numbers.
def make_even(nums):
for i in range(len(nums)):
if nums[i] % 2 == 0:
# If the num is already even we jump to the next iteration using continue
continue
# Only odd numbers will get to this point
# We double the number so it becomes even
nums[i] *= 2
Hopefully, by understanding the break and continue statements, you managed to differentiate them from the pass statement.
Best Practices for Using the Pass Statement
✅ Remember the pass statement is ordinarily used as a temporary placeholder; remember to replace the pass statement with the actual code when ready.
✅ If you use the pass statement for anything other than as a temporary placeholder, include a comment explaining why it is there.
Final Words
In this article, I explained the pass statement in Python, a useful keyword that enables you to divide and conquer when writing code. The pass statement is also covered in Python’s documentation.
Next, check out the common Python error types and how to resolve them.