One of the great perks of using Python is its simplicity. It is easy to work with it because its standard library has many helpful functions. One such function is the sorted function.
This function is used to sort iterables by some order. Without such a function, one must write code implementing a sorting algorithm such as Bubble Sort or Insertion Sort. This is often hard, but Python provides a simpler way, which we will cover in this article.
Introduction to the Sorted Function
The sorted function is a function that sorts iterables in Python. An iterable is any value that you can loop over. Examples of iterables include strings, lists, tuples, and sets. These iterables are often unordered, and sorting puts their values in some specified order. Ordering values is helpful because:
- Searching with values is faster and more efficient using algorithms such as Binary Search. However, binary search requires the values to be sorted first.
- To display values. At times users would like to view information sorted, for example, the lowest price first or the most recent post first. This would require implementing some way of sorting a list of values.
- When performing statistical analysis, for example, finding the most frequently occurring value in a set. It is easier to do this when the values are sorted in order.
Sorted Function Usage Guide
As mentioned earlier, the sorted function works with all iterables. In turn, it returns a list that has been sorted. This is important to note – while the input can be any iterable, the return value must always be a list.
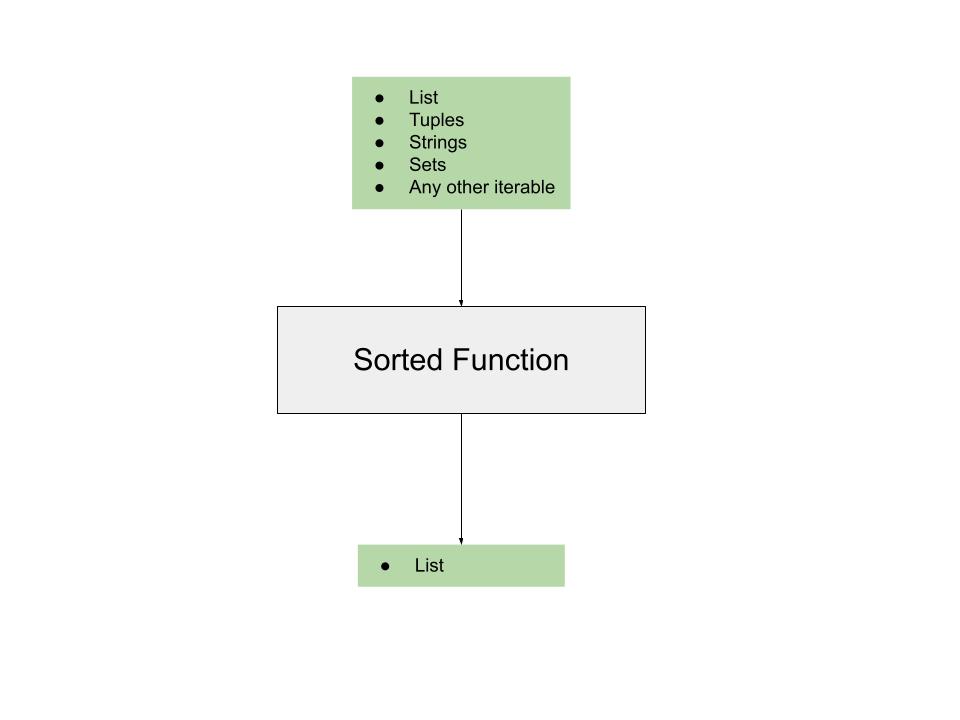
Syntax of Sorted Function
The function signature of the sorted function is as follows:
sorted(iterable, key=None, reverse=False)
As you can see, the only required argument is the iterable, which will be sorted.
The following argument is the key. The key is a function that will be used to transform each element in the iterable to obtain a value that will be used for sorting. This will be useful for sorting a list of dictionaries, as you will see later. The default value is none, so it won’t apply any function unless specified.
The last argument is the reverse argument. When set to true, items will be sorted in reverse order.
In the next section, I will use examples to demonstrate how to use the function.
Sorted Function Usage Examples
List of Numbers
The simplest case of sorting values is sorting a list of numbers. Consider the following code example:
# A list of unsorted values
numbers = [8, 4, 3, 9, 2, 0, 3]
# Sorting the numbers
sorted_numbers = sorted(numbers)
# Outputting the sorted values
print(sorted_numbers)
The output would be:
[0, 2, 3, 3, 4, 8, 9]
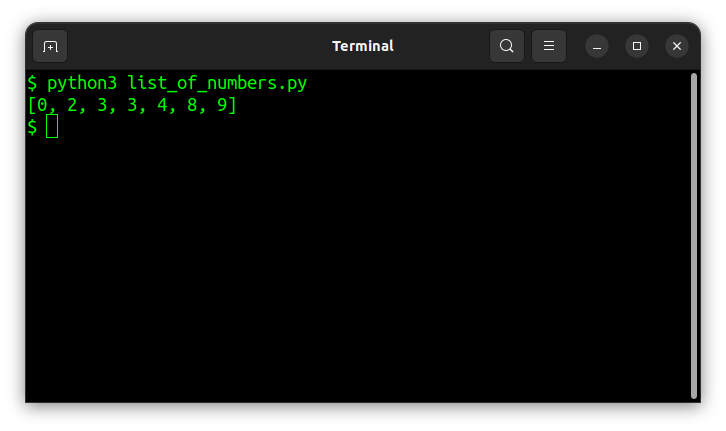
As you can see, the values have been sorted in ascending order. You would set reverse to true if you wanted to sort them in descending order. Therefore line 4 in the previous code example would be:
sorted_numbers = sorted(numbers, reverse=True)
The output of running the modified program would be:
[9, 8, 4, 3, 3, 2, 0]
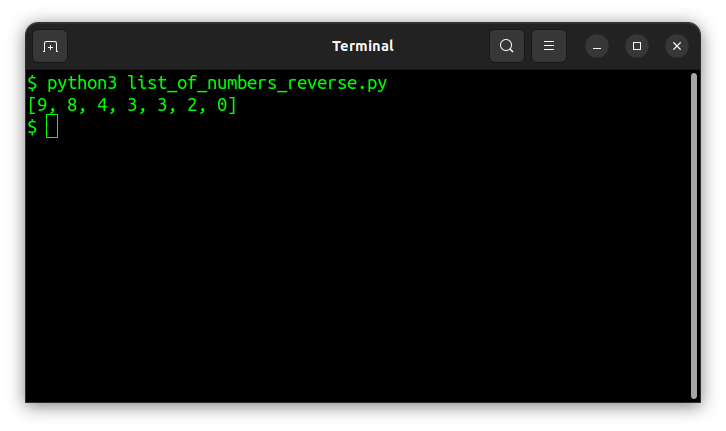
List of Strings
The sorted function supports more than just numbers. You can also sort strings. To sort strings in a list, the first characters of the strings are compared. The comparisons are performed on the characters’ ASCII values. For example, ‘hello’ would come before the word ‘world’ because the ASCII value of ‘h’ is 104, less than the ASCII value of ‘w’, 119.
If one or more strings have the same first character, their second and subsequent characters are compared until some order is found. Here is a code example where we sort people’s names.
# Creating a list of names
members_list = ['bob', 'dave', 'charlie', 'alice']
# Sorting the names
sorted_members_list = sorted(members_list)
# Printing the names
print(sorted_members_list)
This will produce the following output:
['alice', 'bob', 'charlie', 'dave']
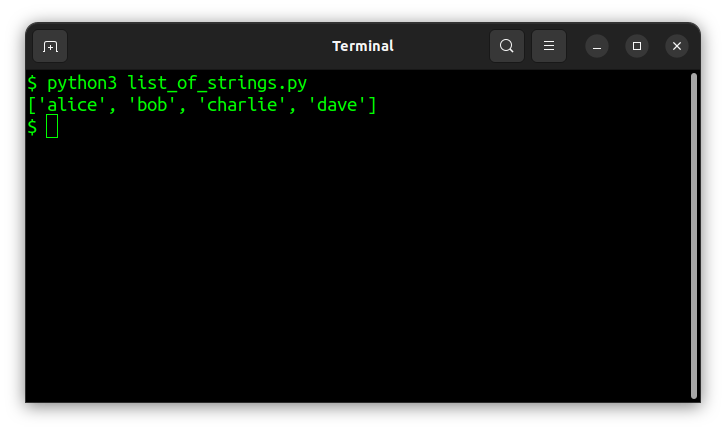
Because ASCII values are used, the ordering of strings depends on which character comes first in the ASCII table. For example, an uppercase character would come before a lowercase one because uppercase characters come before lowercase letters in ASCII. Here’s a complete ASCII table for your reference:
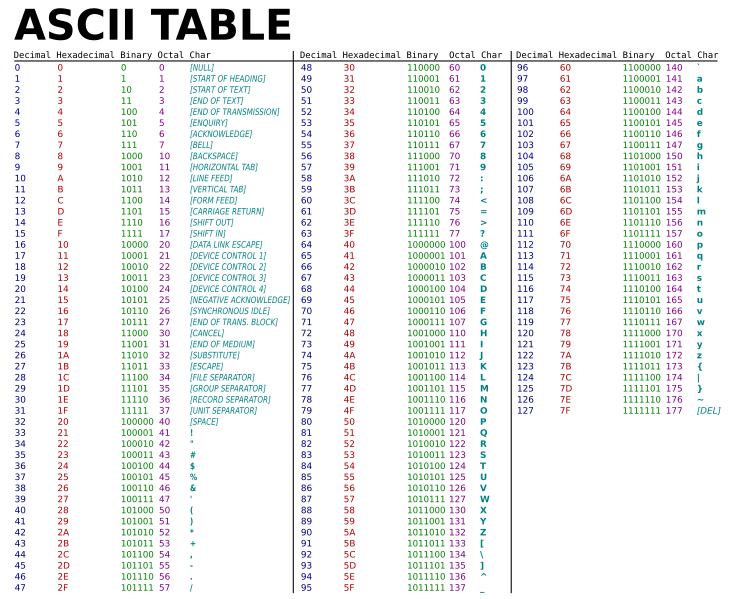
Other Iterables – Strings, Tuples, and Sets
As I mentioned, the sorted function works with all sorts of iterables. The same rules apply to how the values in the iterables will be sorted. Here is an example:
# Printing a sorted string
print(sorted("dijkstra"))
# Printing a sorted tuple of values
print(sorted((3, 4, 2, 1, 5, 0)))
# Printing a sorted set of values
print(sorted(set([4, 5, 5, 1, 3, 8, 9])))
The output of this will be:
['a', 'd', 'i', 'j', 'k', 'r', 's', 't']
[0, 1, 2, 3, 4, 5]
[1, 3, 4, 5, 8, 9]
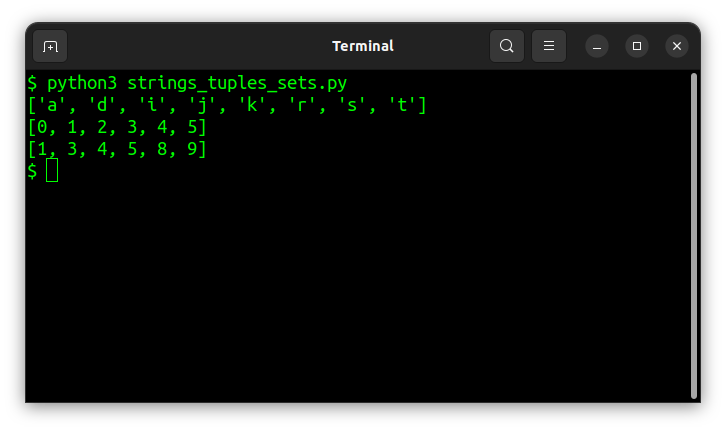
As you can see, the output in each case is a list.
List of Dictionaries
You can also apply the sorted function to sort a list of dictionaries. However, sorting dictionaries is a bit more complicated. This is because, unlike numbers or strings, a dictionary has multiple properties, each equally valid for comparison.
So to sort dictionaries, you specify a function that will summarise the entire dictionary to one value that will be used for comparison. This function will be passed to the sorted function as the key argument. Here’s an example to illustrate:
people = [
{ 'name': 'Alice', 'age': 27 },
{ 'name': 'Bob', 'age': 23 },
{ 'name': 'Charlie', 'age': 25}
]
people_sorted_by_age = sorted(people, key=lambda person: person['age'])
print(people_sorted_by_age)
In this example, we have three people represented by a dictionary object. Each object has a name and age attribute. We want to sort people by age. Therefore when we call the sorted function, we pass in a function as the key argument.
This function will take in a person’s dictionary object and return the person’s age. The return value of this key will be used for sorting. Therefore the entire dictionary has been summarised into a simple integer that can be compared. For simplicity, I have used a lambda function to define the key argument.
Running the code will produce the following output:
[{'name': 'Bob', 'age': 23}, {'name': 'Charlie', 'age': 25}, {'name': 'Alice', 'age': 27}]
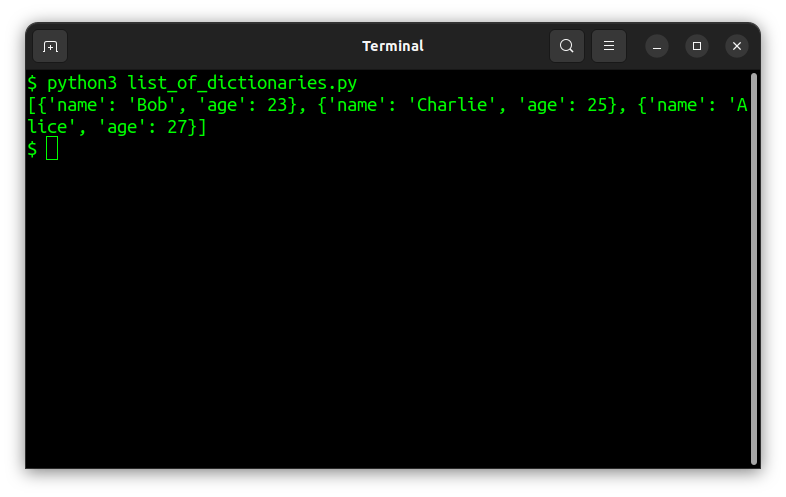
Key Argument Use Case
The key argument does not have to be used when sorting dictionaries only. You can use it on all values. Its use is to provide a key that can be used to sort values. Here are example use cases:
- Sorting values through defining a key function that takes in the value and returns the length of the value.
- Sorting strings in a list in a case-insensitive manner. To do this, each string in the list can be converted to lowercase. This can be achieved by defining a key function that takes in the key value of a string and returns a lowercase version of the string.
- Sorting values based on a composite value that combines the values of other entries.
Runtime Complexity of the Sorted Function
The sorted function has a runtime complexity of O(n log n), where n is the number of elements in the input iterable. This complexity arises because the function uses the Timsort algorithm, which is a hybrid sorting algorithm based on merge sort and insertion sort.
The space complexity of the function is O(n), where n is still the number of elements in the input. This is because a new list is created and returned.
Sorted Function vs. Sort Function
Another option for sorting values is the sort function. This section will explain the key differences between the sorted and sort functions.
- The sort function modifies the iterable in place, while the sorted function creates a new list and returns that.
- Because the modifications are carried out in place, sort requires that the input be a list. On the other hand, sorted can take any iterable as input which will then be used to create a new list that will be modified and returned.
Final Words
In this article, we covered the sorted function – what it is, how to use it, and the different arguments it takes in. We also covered different usage examples of the function and its runtime complexity and compared it to the sort function.
Next, you may want to read our article on Python’s Sum Function.